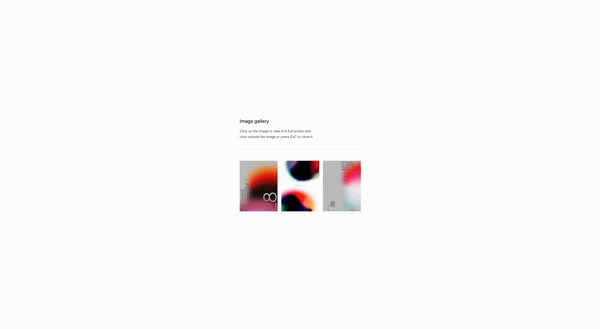
Today we are building a simple image gallery using Tailwind CSS and JavaScript. Just like the one we built in the previous tutorial, with Alpine JS, but this time we will use vainilla JS.
What are image galleries?
Image galleries serve as a method to present a collection of images in an attractive manner. They are employed to display various images or to offer a preview of a product or service.
Use Cases:
- Product images: For instance, a product gallery can display multiple images or features of a product.
- Service images: A service gallery can highlight different images or features of a service.
- Event images: An event gallery can present various images or features of an event.
- Blog images: A blog gallery can feature multiple images or highlights from blog posts.
These are just a few examples. There are numerous applications for image galleries, with endless possibilities.
Let’s write the html
Understanding the code
ìd="image-gallery"
: This is the id of the container element that will hold the image gallery.data-image-url="..."
: This is a data attribute that will be used to store the URL of the image that will be displayed in the gallery.id="modal"
: This is the id of the modal element that will be used to display the image when clicked.id="modal-content"
: This is the id of the modal content element that will be used to display the image.id="modal-close-button"
: This is the id of the modal close button element that will be used to close the modal.id="modal-image"
: This is the id of the modal image element that will be used to display the image.
<div id="image-gallery">
<div>
<div data-image-url="...">
<img src="..." />
</div>
<!-- Add more image placeholders as needed -->
</div>
<!-- Modal -->
<div id="modal">
<div id="modal-content">
<button id="modal-close-button">Close</button>
<img id="modal-image" />
</div>
</div>
</div>
Let’s write the JavaScript
document.addEventListener("DOMContentLoaded", function () {
: This is the event listener that will be triggered when the DOM is fully loaded.const gallery = document.getElementById("image-gallery");
: This is the code that will select theimage-gallery
element and store it in a variable calledgallery
.const modal = document.getElementById("modal");
: This is the code that will select themodal
element and store it in a variable calledmodal
.const modalImage = document.getElementById("modal-image");
: This is the code that will select themodal-image
element and store it in a variable calledmodalImage
.const closeModalButton = document.getElementById("modal-close-button");
: This is the code that will select themodal-close-button
element and store it in a variable calledcloseModalButton
.
The gallery
gallery.addEventListener("click", function (event) {
: This is the event listener that will be triggered when theimage-gallery
element is clicked.if (event.target.tagName === "IMG") {
: This is the code that will check if the clicked element is animg
element.const imageUrl = event.target.parentElement.getAttribute("data-image-url");
: This is the code that will get thedata-image-url
attribute of the clicked element’s parent element and store it in a variable calledimageUrl
.modalImage.src = imageUrl;
: This is the code that will set thesrc
attribute of themodal-image
element to theimageUrl
variable.modal.classList.remove("hidden");
: This is the code that will remove thehidden
class from themodal
element.modal.classList.add("flex", "flex-col");
: This is the code that will add theflex
andflex-col
classes to themodal
element.
The modal
function closeModal() {
: This is the function that will be called when themodal-close-button
is clicked.modal.classList.add("hidden");
: This is the code that will add thehidden
class to themodal
element.modal.classList.remove("flex", "flex-col");
: This is the code that will remove theflex
andflex-col
classes from themodal
element.
Closing the modal
closeModalButton.addEventListener("click", closeModal);
: This is the event listener that will be triggered when themodal-close-button
is clicked.document.addEventListener("keydown", function (event) {
: This is the event listener that will be triggered when a key is pressed.if (event.key === "Escape") {
: This is the code that will check if the pressed key isEscape
.closeModal();
: This is the code that will call thecloseModal
function.
document.addEventListener("DOMContentLoaded", function () {
const gallery = document.getElementById("image-gallery");
const modal = document.getElementById("modal");
const modalImage = document.getElementById("modal-image");
const closeModalButton = document.getElementById("modal-close-button");
gallery.addEventListener("click", function (event) {
if (event.target.tagName === "IMG") {
const imageUrl =
event.target.parentElement.getAttribute("data-image-url");
modalImage.src = imageUrl;
modal.classList.remove("hidden");
modal.classList.add("flex", "flex-col");
}
});
function closeModal() {
modal.classList.add("hidden");
modal.classList.remove("flex", "flex-col");
}
modal.addEventListener("click", function (event) {
if (
event.target.id === "modal" ||
event.target.id === "modal-close-button"
) {
closeModal();
}
});
closeModalButton.addEventListener("click", closeModal);
document.addEventListener("keydown", function (event) {
if (event.key === "Escape") {
closeModal();
}
});
});
Conclusion
In this tutorial, we learned how to create a simple image gallery using Tailwind CSS and JavaScript. We covered the basics of HTML and the JavaScript code that will be used to create the gallery. You can style it with CSS or however you like it, we chose Tailwind CSS for this tutorial.
Hope you found this tutorial helpful and have a nice day!
/Michael Andreuzza
One price.
Lifetime access.
-
34 Premium Astro Templates
-
All Future Templates Included
-
Unlimited Projects · Lifetime License




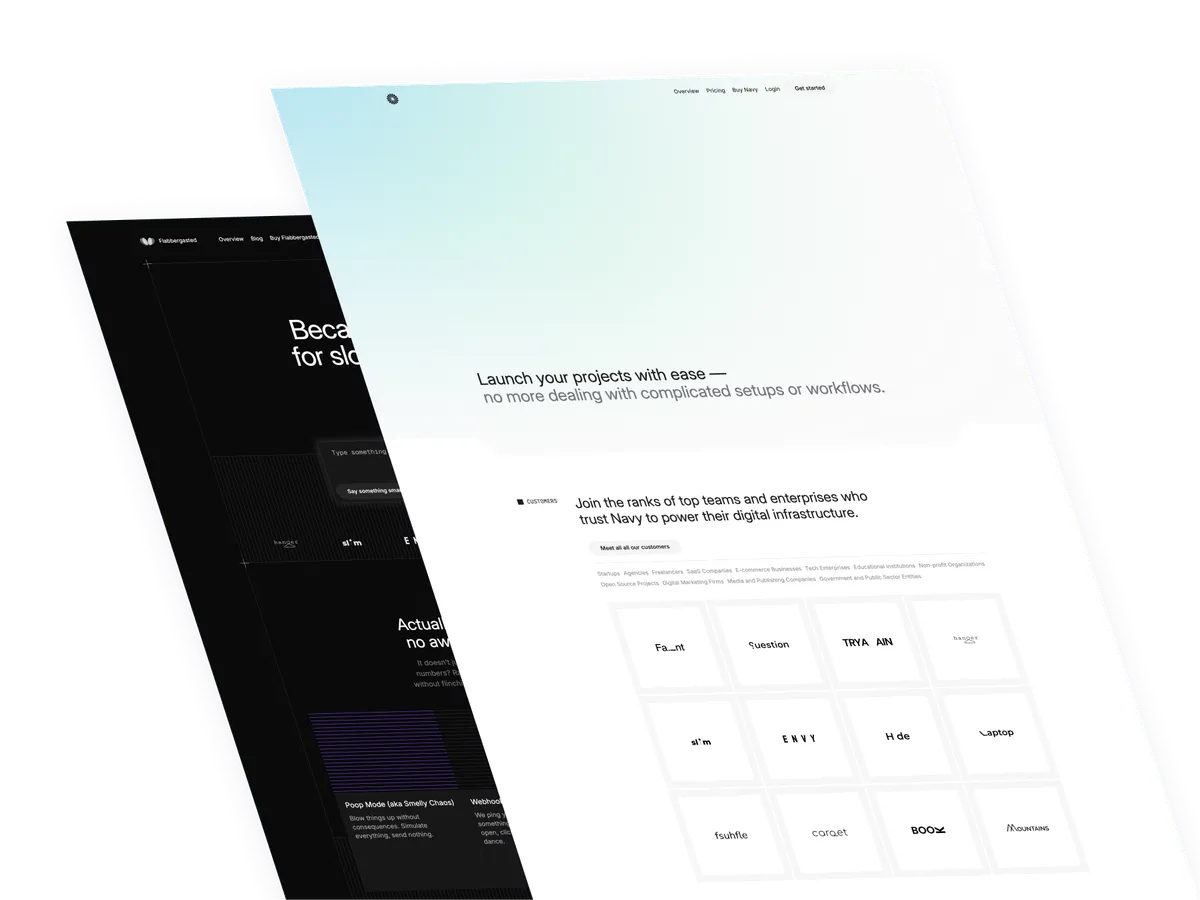