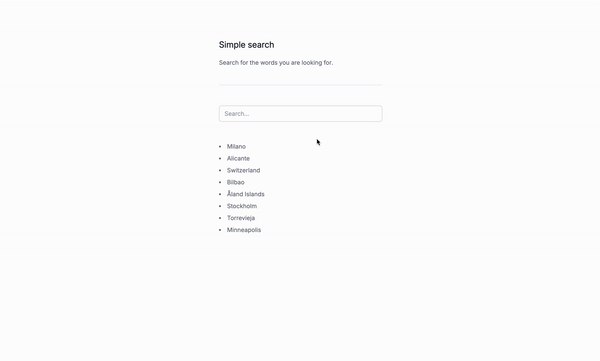
Today we’re going to create a search input with Tailwind CSS and JavaScript. Just like the previous tutorial with Alpinejs, we’ll use the same structure and we’ll add some JavaScript to make it interactive.
What is a Search Input?
A search input is a form control that allows users to enter a search query and retrieve relevant results from a database or search engine. It is a crucial component in web applications, enabling users to find specific content or information quickly and efficiently. The search input is typically accompanied by a search button or icon to initiate the search process. In some cases, the search may start automatically as the user types in their query, providing instant feedback and results.
Key Features:
- Interactive: Users can type in queries and get real-time suggestions or results.
- Versatile: Can be used in various applications, from e-commerce sites to file management systems.
- User-friendly: Simplifies the process of finding specific items or information.
Use Cases:
- Product Search:
- A search input can be integrated into an e-commerce site to help users find products in a vast inventory. For example, users can type in the name of a product, and the search input will filter and display matching items.
- File Search:
- In file management systems or operating systems, a search input can help users locate files within directories or the entire file system. This is particularly useful for quickly finding documents, images, or any other type of file.
- Document Search:
- Search inputs are essential in document management systems, allowing users to search through a large database of documents. This functionality is beneficial for organizations that need to manage and retrieve documents efficiently.
- Data Search:
- In applications dealing with large datasets, a search input enables users to find specific data points within a database or search engine. This is crucial for data analysis, reporting, and decision-making processes.
Let’s build the markup
Understanding the code:
id="search-component"
: This is the container for the search input and the list of items.id="search-input"
: This is the search input.id="items-list"
: This is the list of items that will be filtered based on the search query.
Classes are omited for brevity
<div id="search-component">
<!-- Search Input -->
<input id="search-input" />
<!-- Filtered Items -->
<ul id="items-list">
<!-- List items will be injected here by JavaScript -->
</ul>
</div>
Let’s add some JavaScript
The addEventListener method
document.addEventListener("DOMContentLoaded", function() {})
: This is the event listener that will be triggered when the DOM (Document Object Model) is fully loaded and ready for interaction.const searchComponent = document.getElementById("search-component");
: This is the search component element that we want to interact with.const searchInput = document.getElementById("search-input");
: This is the search input element that we want to interact with.const itemsList = document.getElementById("items-list");
: This is the list of items that we want to interact with.
The items array
const items = ["Milano", "Alicante", "Switzerland", "Bilbao", "Åland Islands", "Stockholm", "Torrevieja", "Minneapolis"];
: This is an array of items that we want to filter based on the search query.
Rendering items
function renderItems(filter = "") {
: This is a function that will be called when the search input value changes.itemsList.innerHTML = "";
: This is a line of code that will clear the list of items.const filteredItems = items.filter((item) => item.toLowerCase().includes(filter.toLowerCase()));
: This is a line of code that will filter the items based on the search query.filteredItems.forEach((item) => {
: This is a line of code that will iterate over the filtered items and create a new list item for each item.const li = document.createElement("li");
: This is a line of code that will create a new list item element.li.textContent = item;
: This is a line of code that will set the text content of the list item.itemsList.appendChild(li);
: This is a line of code that will append the list item to the list of items.
Adding event listener
searchInput.addEventListener("input", function() {
: This is a line of code that will add an event listener to the search input.renderItems(searchInput.value);
: This is a line of code that will call the renderItems function with the search input value as an argument.
Initial render
renderItems();
: This is a line of code that will call the renderItems function without any arguments.
document.addEventListener("DOMContentLoaded", function () {
const searchComponent = document.getElementById("search-component");
const searchInput = document.getElementById("search-input");
const itemsList = document.getElementById("items-list");
const items = [
"Milano",
"Alicante",
"Switzerland",
"Bilbao",
"Åland Islands",
"Stockholm",
"Torrevieja",
"Minneapolis",
];
function renderItems(filter = "") {
itemsList.innerHTML = "";
const filteredItems = items.filter((item) =>
item.toLowerCase().includes(filter.toLowerCase())
);
filteredItems.forEach((item) => {
const li = document.createElement("li");
li.textContent = item;
itemsList.appendChild(li);
});
}
searchInput.addEventListener("input", function () {
renderItems(searchInput.value);
});
// Initial render
renderItems();
});
Conclusion
This is a super simple Search that can be used to filter a list of items based on a search query without the need for any additional libraries or frameworks. This is not for production use, but it’s a great way to learn how to build interactive user interfaces with JavaScript.
Hope you enjoyed this tutorial and have a great day!
/Michael Andreuzza
Unlock all themes for $149 and own them forever! Includes lifetime updates, new themes, unlimited projects, and support
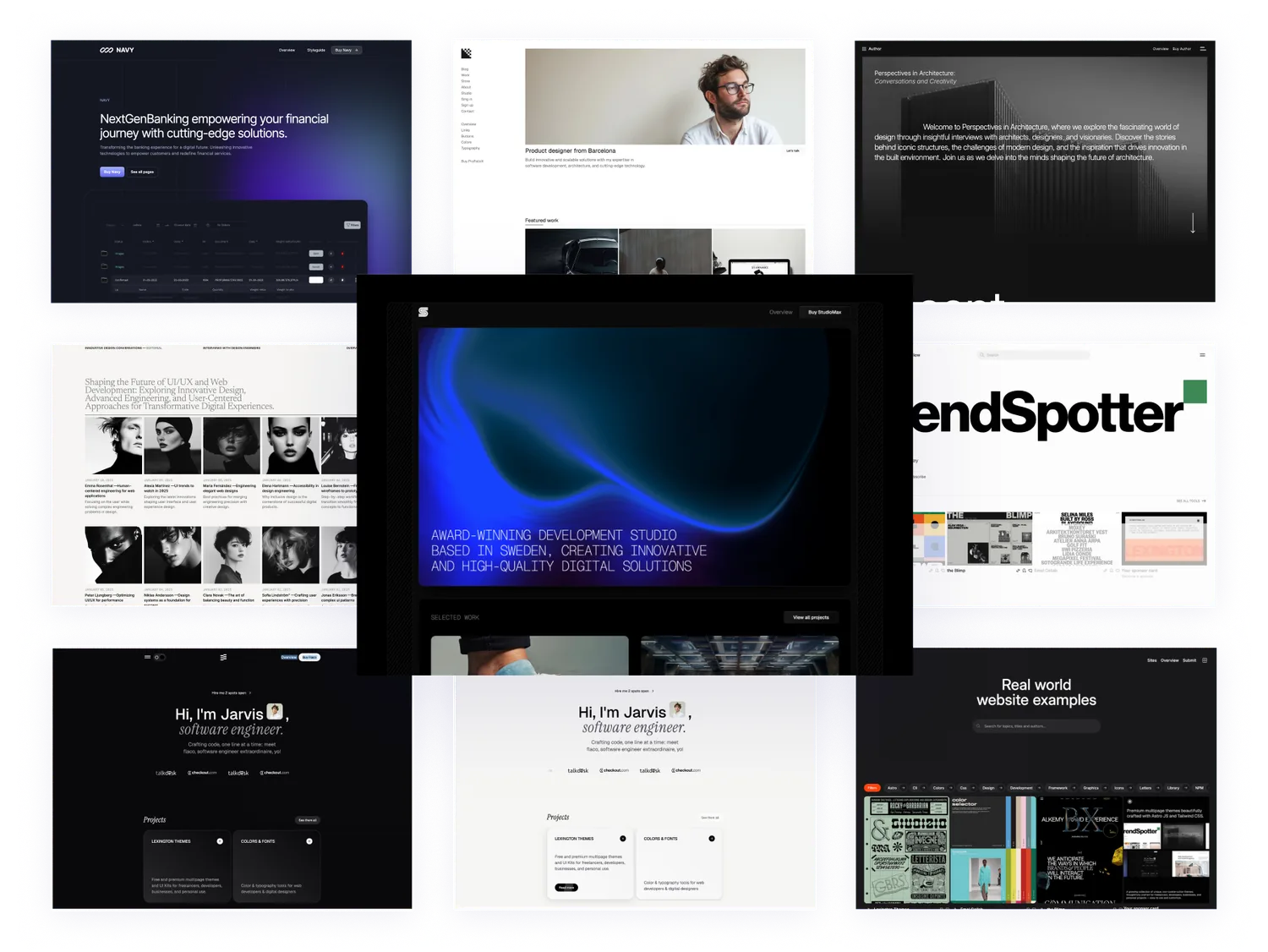