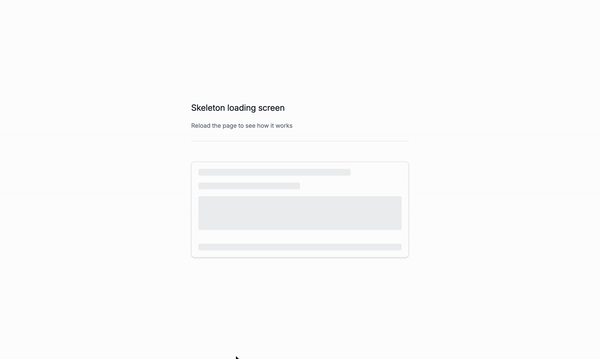
It’s Monday again, yay! Today, we’re going to build a skeleton loading screen using JavaScript and Tailwind CSS. Dang, aren’t we fancy?
What are skeleton loaders?
A skeleton loader is a loading screen that appears while content is being loaded. It’s a visual representation of the content that’s being loaded, giving the user a sense of progress and a better understanding of what’s happening. Skeleton loaders are commonly used in web development to provide a more polished and professional look to the user interface.
Use cases
Skeleton loaders are useful in a variety of scenarios, including:
- Loading content: When fetching data from an API or loading a large amount of data, a skeleton loader can provide a visual representation of the content being loaded, giving the user a sense of progress.
- Loading states: When a user is interacting with a form or a page, a skeleton loader can be used to provide a loading state, giving the user a better understanding of what’s happening.
- Empty states: When a user has not yet interacted with a page or a feature, a skeleton loader can be used to provide a visual representation of an empty state, giving the user a sense of progress.
- Placeholders: When a user is searching for something, a skeleton loader can be used to provide a placeholder for the search results, giving the user a better understanding of what’s happening.
and many other similars use cases.
Lets get to the markup
The skeleton loader
Id’s
id="content"
: Assigns a unique ID to the content element.
<div id="content">
<!-- Skeleton loader will be here -->
</div>
The skeleton
The skeleton is made with a series of divs with different widths and heights without content. It’s a visual representation of the content that’s being loaded.
<div class="p-4 animate-pulse space-y-4">
<div class="w-3/4 h-4 rounded bg-base-200"></div>
<div class="w-1/2 h-4 rounded bg-base-200"></div>
<div class="h-20 rounded bg-base-200"></div>
<div class="flex flex-col gap-2">
<div class="w-5/6 h-4 rounded bg-base-200"></div>
<div class="w-4/6 h-4 rounded bg-base-200"></div>
</div>
</div>
The actual content
The actual content is the content that the customr will be consuming, using, etc,…
<div class="p-4 prose">
<h4>Actual Content</h4>
<p>This is the real content that has finished loading.</p>
<img src="https://i.pinimg.com/564x/a4/ae/54/a4ae54bdd21cbbdb2ccc377ab07b6c6f.jpg" alt="Placeholder" class="w-full mt-4 rounded">
<p>Additional text content goes here. It can be as long as needed.</p>
</div>
Lets get to the script
The createSkeletonLoader function
The createSkeletonLoader function
function createSkeletonLoader()
: This function creates the skeleton loader markup.return
: This line of code returns the skeleton loader markup.return
: This line of code returns the skeleton loader markup. The createContent functionfunction createContent()
: This function creates the actual content markup.return
: This line of code returns the actual content markup. The contentDiv variableconst contentDiv = document.getElementById("content");
: This line of code selects the content element with the IDcontent
. Showing the skeleton loadercontentDiv.innerHTML = createSkeletonLoader();
: This line of code sets the inner HTML of the content element to the skeleton loader markup. Simulating content loadingsetTimeout(() => {
: This line of code sets a timeout function that will be executed after 2 seconds.contentDiv.innerHTML = createContent();
: This line of code sets the inner HTML of the content element to the actual content markup.}, 2000);
: This line of code sets the timeout duration to 2 seconds.
function createSkeletonLoader() {
return `
<div class="p-4 animate-pulse space-y-4">
<div class="w-3/4 h-4 rounded bg-base-200"></div>
<div class="w-1/2 h-4 rounded bg-base-200"></div>
<div class="h-20 rounded bg-base-200 "></div>
<div class="flex flex-col gap-2>
<div class="w-5/6 h-4 rounded bg-base-200"></div>
<div class="w-4/6 h-4 rounded bg-base-200"></div>
</div>
</div>
`;
}
function createContent() {
return `
<div class="p-4 prose">
<h4>Actual Content</h4>
<p>This is the real content that has finished loading.</p>
<img src="https://i.pinimg.com/564x/a4/ae/54/a4ae54bdd21cbbdb2ccc377ab07b6c6f.jpg" alt="Placeholder" class="w-full mt-4 rounded">
<p>Additional text content goes here. It can be as long as needed.</p>
</div>
`;
}
const contentDiv = document.getElementById("content");
// Show skeleton loader
contentDiv.innerHTML = createSkeletonLoader();
// Simulate content loading
setTimeout(() => {
contentDiv.innerHTML = createContent();
}, 2000); // Change content after 2 seconds
Conclusion
In this tutorial, we learned how to create a skeleton loading screen using JavaScript and Tailwind CSS. We saw how to create a skeleton loader and an actual content section, and how to show and hide them using JavaScript.
I hope you found this tutorial helpful and have a great day!
/Michael Andreuzza