How to create a typewriter effect text animation with Tailwind CSS and JavaScript
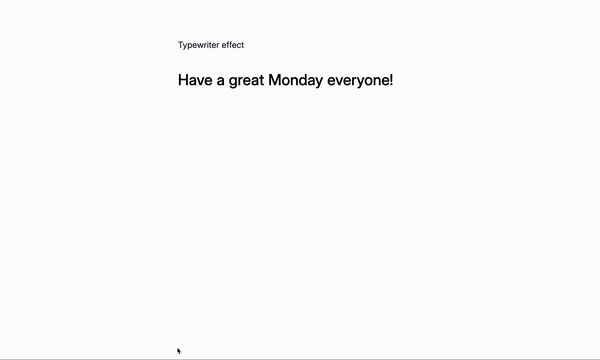
Hello everyone, today we are going to create a super simple typewriter effect text animation with Tailwind CSS and JavaScript.
What is a typewriter effect?
A typewriter effect is a text animation where characters appear one by one, simulating the look of text being typed out on a typewriter. It’s a visually engaging way to introduce text content in a dynamic fashion, often used in hero sections, introductions, or storytelling elements.
Use cases
- Landing pages: Highlight key messages or product features.
- Presentations: Create a dramatic introduction or reveal key points.
- Storytelling: Enhance narrative content with a touch of nostalgia.
- Portfolio sites: Show your creative flair by animating project descriptions or titles.
- Loading screens: Keep users engaged while waiting for content to load.
Let’s get started
Firt, we need to create a paragraph element with the id of “typewriter”. ID’s
id="typewriter"
: This is the id of the paragraph element that will contain the text. This will be the element that will be animated.
<p id="typewriter">
<!-- The text will be inserted here by JavaScript -->
</p>
The Script
Creating the variables
const text = "Have a great Monday everyone!";
: This line of code will define the text that will be animated. In this case, it’s a simple string that says “Have a great Monday everyone!”.const typewriter = document.getElementById("typewriter");
: This line of code will get the element with the id of “typewriter” and store it in a variable calledtypewriter
.let i = 0;
: This line of code will define a variable calledi
and set it to 0.
The typewriter function
function typeWrite()
: This line of code will define a function calledtypeWrite
that will be called when the page is loaded.if (i < text.length)
: This line of code will check if the value ofi
is less than the length of thetext
string.typewriter.innerHTML += text.charAt(i);
: This line of code will add the character at indexi
of thetext
string to thetypewriter
element.i++;
: This line of code will increment the value ofi
by 1.setTimeout(typeWrite, 100);
: This line of code will set a timeout of 100 milliseconds (0.1 seconds) and call thetypeWrite
function.} else {
: This line of code will be executed if the conditioni < text.length
is false.setTimeout(resetTypewriter, 2000);
: This line of code will set a timeout of 2000 milliseconds (2 seconds) and call theresetTypewriter
function.
The resetTypewriter function
function resetTypewriter()
: This line of code will define a function calledresetTypewriter
that will be called when the timeout is complete.typewriter.innerHTML = "";
: This line of code will clear the content of thetypewriter
element.i = 0;
: This line of code will set the value ofi
to 0.
Calling the functions
typeWrite();
: This line of code will call thetypeWrite
function.
const text = "Have a great Monday everyone!";
const typewriter = document.getElementById("typewriter");
let i = 0;
function typeWrite() {
if (i < text.length) {
typewriter.innerHTML += text.charAt(i);
i++;
setTimeout(typeWrite, 100);
} else {
setTimeout(resetTypewriter, 2000);
}
}
function resetTypewriter() {
typewriter.innerHTML = "";
i = 0;
typeWrite();
}
typeWrite();
Conclusion
This is a simple typewriter effect text animation that you can recreate with Tailwind CSS and JavaScript. You can customize the text and style to fit your needs. Before using it in your project, make sure to test it thoroughly to ensure that it works as expected and it’s accessible to all users.
Fun fact: You can also do this wit only CSS and/or Tailwind CSS, so need need to overengineer your project.
Hope you enjoyed this tutorial and have a good day!
/Michael Andreuzza
One Price.
Lifetime Access.
-
33 Premium Astro Templates
-
All Future Templates Included
-
Unlimited Projects · Lifetime License




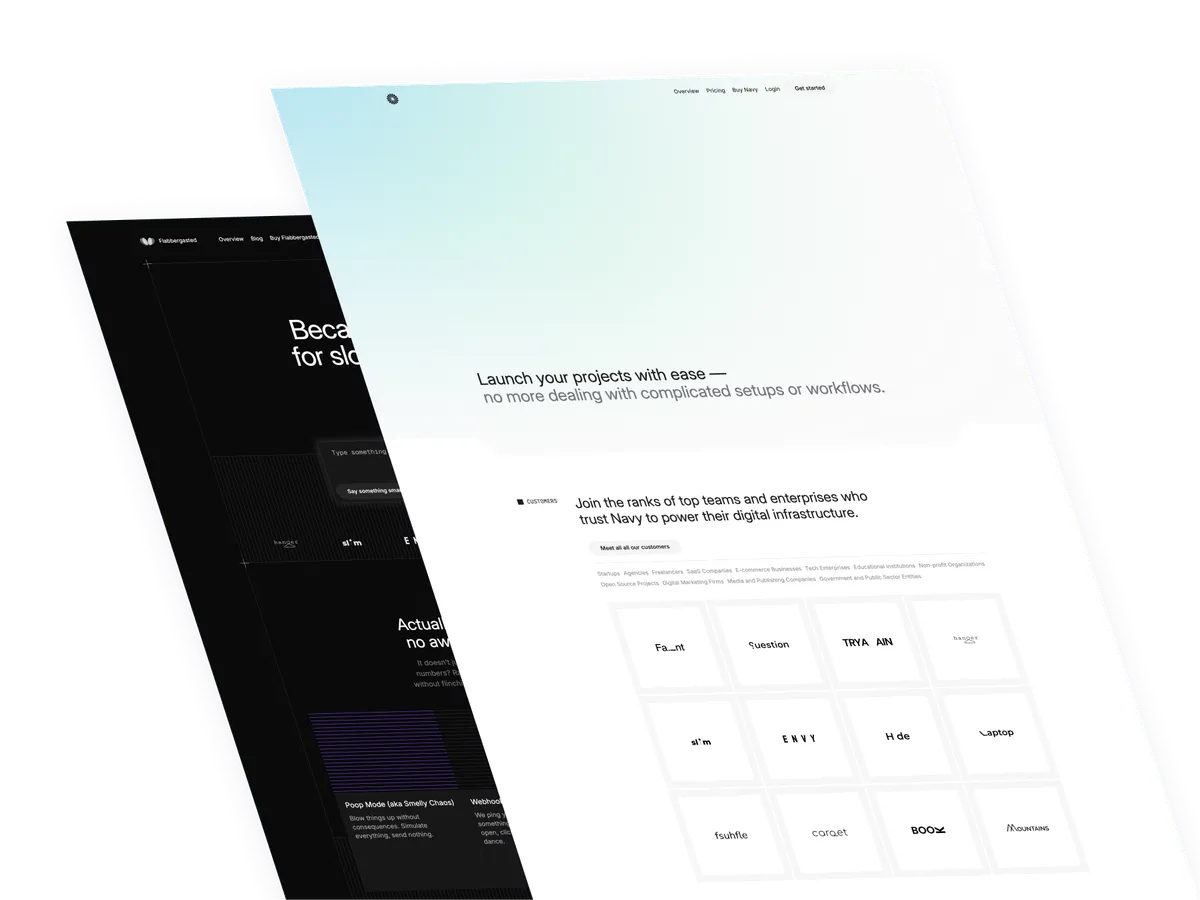